- Home
- Premium Memberships
- Lottery Results
- Forums
- Predictions
- Lottery Post Videos
- News
- Search Drawings
- Search Lottery Post
- Lottery Systems
- Lottery Charts
- Lottery Wheels
- Worldwide Jackpots
- Quick Picks
- On This Day in History
- Blogs
- Online Games
- Premium Features
- Contact Us
- Whitelist Lottery Post
- Rules
- Lottery Book Store
- Lottery Post Gift Shop
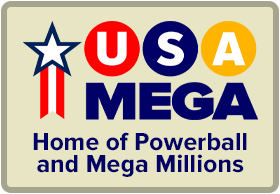
The time is now 5:46 pm
You last visited
May 1, 2024, 4:36 pm
All times shown are
Eastern Time (GMT-5:00)

Generating random arrangement of columns in sets of equal length
Published:
Updated:
Based on the java package from Fermi Labs (randomX) that uses decay of Cæsium-137 particle as the source of random numbers,(hence quantum mechanical physical process for which no known algorithmic explanation exists - extending big thanks to JadeLottery for pointing this out to me!), created this small application to basically take any number of sets and length and create output consisting of sets of the same size but with random column permutations.
Program takes 4 inputs:
iterations - number of times application should run complete full cycle
max - length of set
sets - number of sets
input - 2 dimensional input array
and an one output: (displayed to the console):
output - 2 dimensional randomized output array
Example of an output generated by this application:
Input sets:
06-12-18-31-39-40-43-51-63-71
13-25-34-37-41-55-58-61-77-79
14-21-33-36-38-50-53-62-69-76
Output sets after 10 iterations:
13-12-18-37-41-50-53-51-63-76
06-21-34-31-38-55-58-61-77-71
14-25-33-36-39-40-43-62-69-79
The application could be used to study various strategies as to i.e. measuring how a non-random, pattern-based system predictions compare to predictions made by a truly random process agent.
One could further extend it by assigning weight (bias) to the indvidual number(s) (lock) something I have not implemented keeping implementation to bare minimum.
Example provided will generate randomized pick-10 matrix-set after 10 iterations.
Hope this will be useful to anyone testing their prediction model(s)!
source:
/* Generates random arrangement of columns in sets of equal length */
import randomX.*;
class randomXdemo {
public static void main(String[] args) {
int iterations = 10;
int max = 10;
int sets = 3;
int input[][] = {{6,12,18,31,39,40,43,51,63,71},
{13,25,34,37,41,55,58,61,77,79},
{14,21,33,36,38,50,53,62,69,76}};
/*
int max = 4;
int sets = 6;
int input[][] = {{1, 3, 2, 6},
{3, 9, 1, 5},
{4, 8, 9, 4},
{5, 6, 0, 1},
{6, 4, 8, 3},
{9, 1, 5, 2}};*/
/*
int max = 6;
int sets = 6;
int input[][] = {{3, 12, 14, 33, 49, 51},
{4, 6, 21, 25, 48, 50},
{5, 17, 20, 22, 29, 45},
{10, 18, 23, 42, 43, 55},
{15, 19, 27, 34, 36, 37},
{16, 30, 38, 44, 54, 56}};
*/
int output[][] = new int[sets][max];
display("Input sets:\n\n", input, sets, max);
randomX r = new randomLEcuyer();
for (int n = 0; n < iterations; n++) {
init(output, max, sets);
for (int i = 0; i < max; i++) {
for (int j = 0; j < sets; j++) {
output[find(r, 0, sets, i, output)][i] = input[j][i];
}
}
}
display("Output sets after " + iterations + " iterations:\n\n", output, sets, max);
}
static void init(int output[][], int max, int sets) {
for (int i = 0; i < max; i++) {
for (int j = 0; j < sets; j++)
output[j][i] = -1;
}
}
}
static int find(randomX r, int lBound, int uBound, int i, int output[][]) {
int n = 0;
do {
n = int2range(byte2int(r.nextByte()), lBound, uBound-1);
} while (output[n][i] != -1);
return n;
}
static int int2range(int i, int lBound, int uBound) {
return (int)(i % (uBound-lBound + 1)) + lBound;
}
static int byte2int(byte b) {
if (b < 0)
return (int)b + 0x100;
return b;
}
static void display(String title, int output[][], int sets, int max) {
int n = 0;
StringBuffer sb = new StringBuffer();
sb.append(title);
for (int j = 0; j < sets; j++) {
for (int i = 0; i < max; i++) {
n = output[j][i];
if (n < 10)
sb.append("0");
sb.append(n);
if (i < max-1)
sb.append("-");
}
sb.append("\n");
}
System.out.println(sb.toString());
}
}
/* source end */
Comments
This Blog entry currently has no comments.
Post a Comment
Please Log In
To use this feature you must be logged into your Lottery Post account.
Not a member yet?
If you don't yet have a Lottery Post account, it's simple and free to create one! Just tap the Register button and after a quick process you'll be part of our lottery community.
Register