- Home
- Premium Memberships
- Lottery Results
- Forums
- Predictions
- Lottery Post Videos
- News
- Search Drawings
- Search Lottery Post
- Lottery Systems
- Lottery Charts
- Lottery Wheels
- Worldwide Jackpots
- Quick Picks
- On This Day in History
- Blogs
- Online Games
- Premium Features
- Contact Us
- Whitelist Lottery Post
- Rules
- Lottery Book Store
- Lottery Post Gift Shop
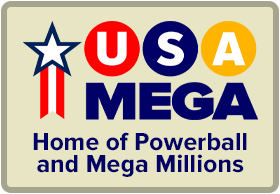
The time is now 2:12 am
You last visited
May 2, 2024, 2:03 am
All times shown are
Eastern Time (GMT-5:00)

Combination Index, VB
Published:
Back on 2003-10-04 I posted a reply that helped find an Index position within a list of ascending order combinations.
As an example in a Pick 6 of 49 Numbers lottery, the first (Index of 1) combination would be (1, 2, 3, 4, 5, 6) and the last (Index of 13,983,816) combination would be (44, 45, 46, 47, 48, 49).
The original reply is linked here -> Lottery Combo Question.
We’ve reworked this a little bit, but would like to post the original here for reference.
Also, now that we have learned C/C++, we have converted it to that language.
Here's the Visual Basic algorithm I've come up with for finding the combinatorial index of a combination for N items taken R at a time.
Those of you who know VB, know what to do.
Those of you who know another programming language and VB should know what to do.
Global Counter(1 To R) As Integer
'R must be assigned some value before program run.
'The program can handle anything R or less when when assigned.
'note: This global array must be put in the declarations part of the function module or some other module.
Function Fact(N) As Double
'The factorial function, Fact(N)= N!
'This is a recursive calling function, means it calls itself multiple times.
'If your BASIC language dose not support recursive calling, then this function needs to be changed to meet the function of:
'N! = N * (N - 1) * (N - 2) * (N - 3) * ... * 3 * 2 * 1, where 0! = 1 and N < 0 is undefined.
If (N < 1) Then
Fact = 1
Else
Fact = N * Fact(N - 1)
End If
End Function
Function Perm(N, R) As Double
'This is the permutational function of N items taken R at a time.
If (N < R) Then
Perm = 0
Else
Perm = Fact(N) / Fact(N - R)
End If
End Function
Function Comb(N, R) As Double
'This is the combinatorial function of N items taken R at a time.
If (N < R) Then
Comb = 0
Else
Comb = Perm(N, R) / Fact(R)
End If
End Function
Function Cdist(N, R, C, Z) As Double
'This is the column distribution function of N items taken R at a time in column C for item Z.
If (Z < C) Or (Z > (N - R + C)) Or (Z > N) Or (C > R) Or (N < 1) Or (R < 1) Or (C < 1) Or (Z < 1) Then
Cdist = 0
Else
Cdist = Comb(Z - 1, C - 1) * Comb(N - Z, R - C)
End If
End Function
Function fColumnSum(N, R, Z) As Double
'This function performs a column count for N items taken R at a time for item Z.
If Z < 1 Then
fColumnSum = 0
ElseIf (Z >= 1) And (Z < N - R + 1) Then
col_sum = 0
For a = 1 To Z
col_sum = col_sum + Cdist(N, R, 1, a)
Next a
fColumnSum = col_sum
ElseIf Z >= N - R + 1 Then
fColumnSum = Comb(N, R)
End If
End Function
Function Comb_Count(N, R) As Double
'This is the combinatorial index for a combination in N items taken R at a time and R must not be larger than the Counter() array size R.
'Counter() is an array containing the combination.
'The Counter() array must be globally dimensioned outside the function and must be the size of R.
'Also, numbers (item index) must be assigned in ascending order; Counter(1)is the minimum number through Counter(R) is the maximum number.
fsum = fColumnSum(N, R, Counter(1) - 1) + 1
For a = 2 To R
fsum = fsum + fColumnSum(N - Counter(a - 1), R - a + 1, Counter(a) - Counter(a - 1) - 1)
Next a
Comb_Count = fsum
End Function
After putting the functions in the appropriate module and putting the Counter array in the declarations section of the main program module, all that needs to be done is assign the combination to the Counter array and call the Comb_Count function.
It would look something like this: (i.e. for a 6/49 lottery with a combination of (06, 10, 17, 37, 40, 49))
Sub Some_subroutine_of_the_main_program()
Counter(1) = 6
Counter(2) = 10
Counter(3) = 17
Counter(4) = 37
Counter(5) = 40
Counter(6) = 49
CombinIndex = Comb_Count(49, 6)
'CombinIndex now contains the combinatorial index of that combination.
End Sub
The combinatorial index of (06, 10, 17, 37, 40, 49) in 49 numbers taken 6 at a time is 7,275,389.
How you want to assign the combination values is up to you.
The way I shown here is just an example.
Also, this only works with single combinations like a 6/49 or 5/35.
Powerball or Mega Millions are not single combos.
They are a multiple combos, a pick 5 part and a pick 1 part.
This algorithm only works on the pick 5 part or the pick 1 part.
You may need to text format some of the code here, however, it will work.
Comments
This Blog entry currently has no comments.
Post a Comment
Please Log In
To use this feature you must be logged into your Lottery Post account.
Not a member yet?
If you don't yet have a Lottery Post account, it's simple and free to create one! Just tap the Register button and after a quick process you'll be part of our lottery community.
Register