- Home
- Premium Memberships
- Lottery Results
- Forums
- Predictions
- Lottery Post Videos
- News
- Search Drawings
- Search Lottery Post
- Lottery Systems
- Lottery Charts
- Lottery Wheels
- Worldwide Jackpots
- Quick Picks
- On This Day in History
- Blogs
- Online Games
- Premium Features
- Contact Us
- Whitelist Lottery Post
- Rules
- Lottery Book Store
- Lottery Post Gift Shop
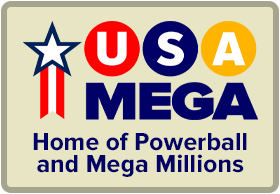
The time is now 12:00 am
You last visited
May 10, 2024, 6:02 pm
All times shown are
Eastern Time (GMT-5:00)

5th Part: "Nostalgia" "Old" Basic Language interpreters, Commodore 64 Basic Interpreter # 1.
Published:
Updated:
Commodore BASIC Character Set
BLANK - separates keywords and variable names
; SEMI-COLON - used in variable lists to format output
= EQUAL SIGN - value assignment and relationship testing
+ PLUS SIGN - arithmetic addition or string concatenation
(concatenation: linking together in a chain)
- MINUS SIGN - arithmetic subtraction, unary minus
* ASTERISK - arithmetic multiplication
/ SLASH - arithmetic division
^ UP ARROW - arithmetic exponentiation
( LEFT PARENTHESIS - expression evaluation and functions
) RIGHT PARENTHESIS - expression evaluation and functions
% PERCENT - declares variable name as an integer
# NUMBER - comes before logical file number in input/
output statements
$ DOLLAR SIGN - declares variable name as a string
, COMMA - used in variable lists to format output; also
separates command parameters
. PERIOD - decimal point in floating point constants
" QUOTATION MARK - encloses string constants
: COLON - separates multiple BASIC statements in a line
? QUESTION MARK - abbreviation for the keyword PRINT
< LESS THAN - used in relationship tests
> GREATER THAN - used in relationship tests
{pi} PI - the numeric constant 3.141592654
-------------------------------------------------------------------------------
Always type NEW and hit <RETURN-ENTER> before starting a new program.
-------------------------------------------------------------------------------
A person should really read the:
COMMODORE 64 PROGRAMMER'S REFERENCE GUIDE
http://www.commodore.ca/manuals/c64_programmers_reference/c64-programmers_reference.htm
http://www.commodore.ca/manuals/c64_users_guide/c64-users_guide.htm
http://project64.c64.org/hw/c64.html
Anyhow read:
Hierarchy of Operations ..................................... 15
And 10 to 17 pages
---------------------------------------------------------------------------
Read about the PRINT and the PRINT# keywords on pages 70 to 76
--------------------
EXPONENTIATION
Use the up arrow (^) to raise a number to a power. Press the <RETURN>
key after you type the calculation. For example, to find 12 to the fifth
power, type this:
PRINT 12^5 Key in this and <RETURN>
248832 The computer displays this
This is the same as:
PRINT 12*12*12*12*12
248832
--------------------
3.3. MULTIPLE CALCULATIONS ON ONE LINE
The last example shows that you can perform more than one calculation
on a line. You can also perform different kinds of calculations on the
same line. For example:
PRINT 3 * 5 - 7 + 2 Key in this and <RETURN>
10 The computer displays this
So far our examples have used small numbers and simple problems. But
the 64 can do much more complex calculations. The next example adds large
numbers.
Notice that 78956.87 doesn't have a comma between the 8 and the 9. You
can't use commas this way in BASIC. BASIC thinks commas indicate new
numbers, so it would think 78,956.87 is two numbers: 78 and 956.87.
Remember to press <RETURN> after you type the problem.
PRINT 1234.5 + 3457.8 + 78956.87
83649.17
The next example uses a ten digit number. The 64 can work with numbers
that have up to ten digits, but can only display nine digits in the
answer. So the 64 rounds numbers that are more than nine digits. Numbers
five and over are rounded up, and numbers four and under are rounded
down. This means that 12123123.45 is rounded to 12123123.5. Because of
rounding, the computer doesn't give the same answer you'd get if you
added these numbers by hand. In this case, the answer is 12131364.817.
You can see the difference rounding makes.
PRINT 12123123.45 + 345.78 + 7895.687
12131364.9
The 64 prints numbers between 0.01 and 999,999,999 using standard
notation, except for leaving out commas in large numbers. Numbers outside
this range are printed using scientific notation. Scientific notation
lets you express a very large or very small number as a power of 10. For
example:
PRINT 123000000000000000
1.23E+17
Another way of expressing this number is 1.23 * 10 ^ 17. The 64 uses
scientific notation for numbers with lots of digits to make them easier
to read.
There is a limit to the numbers the computer can handle, even using
scientific notation. These limits are:
Largest numbers : +/- 1.70141183E+38
Smallest numbers: +/- 2.93873588E-39
3.4. EXECUTION ORDER IN CALCULATIONS
If you tried to perform some mixed calculations of your own, you might
not have gotten the results you expected. This is because the computer
performs calculations in a certain order.
In this calculation:
20 + 8 / 2
the answer is 14 if you add 20 to 8 first, and then divide 28 by 4. But
the answer is 24 if you first divide 8 by 2, and then add 20 and 4.
On the 64, you always get 24 because the computer always performs
calculations in the same order. Problems are solved from left to right,
but within that general movement, some types of calculations take
precedence over others. Here is the order of precedence:
First : - minus sign for negative numbers, not for subtraction.
Second: ^ exponentiation, left to right
Third : * / multiplication and division, left to right
Fourth: + - addition and subtraction, left to right
This means that the computer checks the whole calculation for negative
numbers before doing anything else. Then it looks for exponents; then it
performs all multiplication and division; then it adds and subtracts.
This explains why 20 + 8 / 2 is 24: 8 is divided by 2 before 20 is
added because division has precedence over addition.
There is an easy way to override the order of precedence: enclose any
calculation you want solved first in parentheses. If you add parentheses
to the equation shown above, here's what happens:
PRINT (20 + 8) / 2
14
You get 14 because the parentheses allow 20 and 8 to be added before
the division occurs.
Here's another example that shows how you can change the order, and the
answer, with parentheses:
PRINT 30 + 15 * 2 - 3
57
PRINT (30 + 15) * 2 - 3
87
PRINT 30 + 15 * (2 - 3)
15
PRINT (30 + 15) * (2 - 3)
-45
The last example has two calculations in parentheses. As usual, they're
evaluated from left to right, and then the rest of the problem is solved.
When you have more than one calculation in parentheses, you can further
control the order by using parentheses within parentheses. The problem in
the innermost parentheses is solved first. For example:
PRINT 30 + (15 * (2 - 3))
15
In this case, 3 is subtracted from 2, then 15 is multiplied by -1, and
-15 is added to 30. As you experiment with solving calculations, you'll
get familiar with the order in which mixed calculations are solved.
3.5. COMBINING PRINT'S CAPABILITIES
The 64 computers let you combine the two types of print statements that
you've read about in this book. Remember that anything you enclose in
quotation marks is displayed exactly as you type it.
The next example shows how you can combine the types of PRINT
statements. The equation enclosed in quotes is displayed without being
solved. The equation not in quotes is solved. The semicolon separates the
two parts of the PRINT statement (semicolon means no space).
PRINT "5 * 9 ="; 5 * 9 You key in this and <RETURN>
5 * 9 = 45 The computer displays this
Remember, only the second part of the statement actually solves the
calculation. The two parts are separated by a semicolon. You always have
to separate the parts of a mixed PRINT statement with some punctuation
for it to work the way you want it to. If you use a comma instead of a
semicolon, there is more space between the two parts when they're
displayed. A semicolon leaves out space.
The 64's screen is organized into 4 zones of 10 columns each. When you
use a comma to separate parts of a PRINT statement, the comma works as a
tab, sending each result into the next zone. For example:
PRINT "TOTAL:";95,"SHORTAGE:";15
TOTAL: 95 SHORTAGE: 15
If you have more than four results, they are automatically displayed on
the next line. For example:
PRINT 2 * 3,4 - 6,2 ^ 3,6 / 4,100 + (-48)
6 -2 8 1.5
52
Here's the difference when you use semicolons:
PRINT 2 * 3;4 - 6;2 ^ 3;6 / 4;100 + (-48)
6 -2 8 1.5 52
You can use the difference between the comma and the semicolon in
formatting PRINT statements to create complex displays.
--------------------------------------------------
So next the END KEYWORD
NEW
10 REM How to use the END keyword command
12 PRINT "RED","BROWN","GREEN"
14 END
-----
Copy that and then paste onto the C64 program and then type RUN and hit ENTER
Get:
RED BROWN GREEN
READY.
------------
Also it can work like this:
10 REM How to use the END keyword
12 PRINT"RED":PRINT"BROWN":PRINT"GREEN":END
Copy that and then paste onto the program and then type RUN and hit Enter and the get:
RED
BROWN
GREEN
READY.
-------------
All of that also works on DIRECT Mode, without line numbers.
----------------------------------
Well you already know about the LIST keyword, it will LIST a program loaded into memory into the C64 Interpreter.
----------------------------
If you already have a program listing into the program's memory and want to delete a line for example:
Line 10
10 REM How to use the END keyword command
12 PRINT "RED","BROWN","GREEN"
14 END
Type line 10 without anything on it like this:
10
Then hit the Enter key.
Now list the program's lines:
LIST
And you will see that line 10 is now gone:
12 PRINT "RED","BROWN","GREEN"
14 END
--------------
To change a line for example line 12 type it again:
12 PRINT "RED","BROWN"
14 END
Now if you RUN it you will get:
RED BROWN
READY.
---------------------
Well that is all for today.
Comments
Post a Comment
Please Log In
To use this feature you must be logged into your Lottery Post account.
Not a member yet?
If you don't yet have a Lottery Post account, it's simple and free to create one! Just tap the Register button and after a quick process you'll be part of our lottery community.
Register